Guides
Run Project
- Create an
assets
directory in the project's root directory and copy the configuration.json file from the demo to this directory.
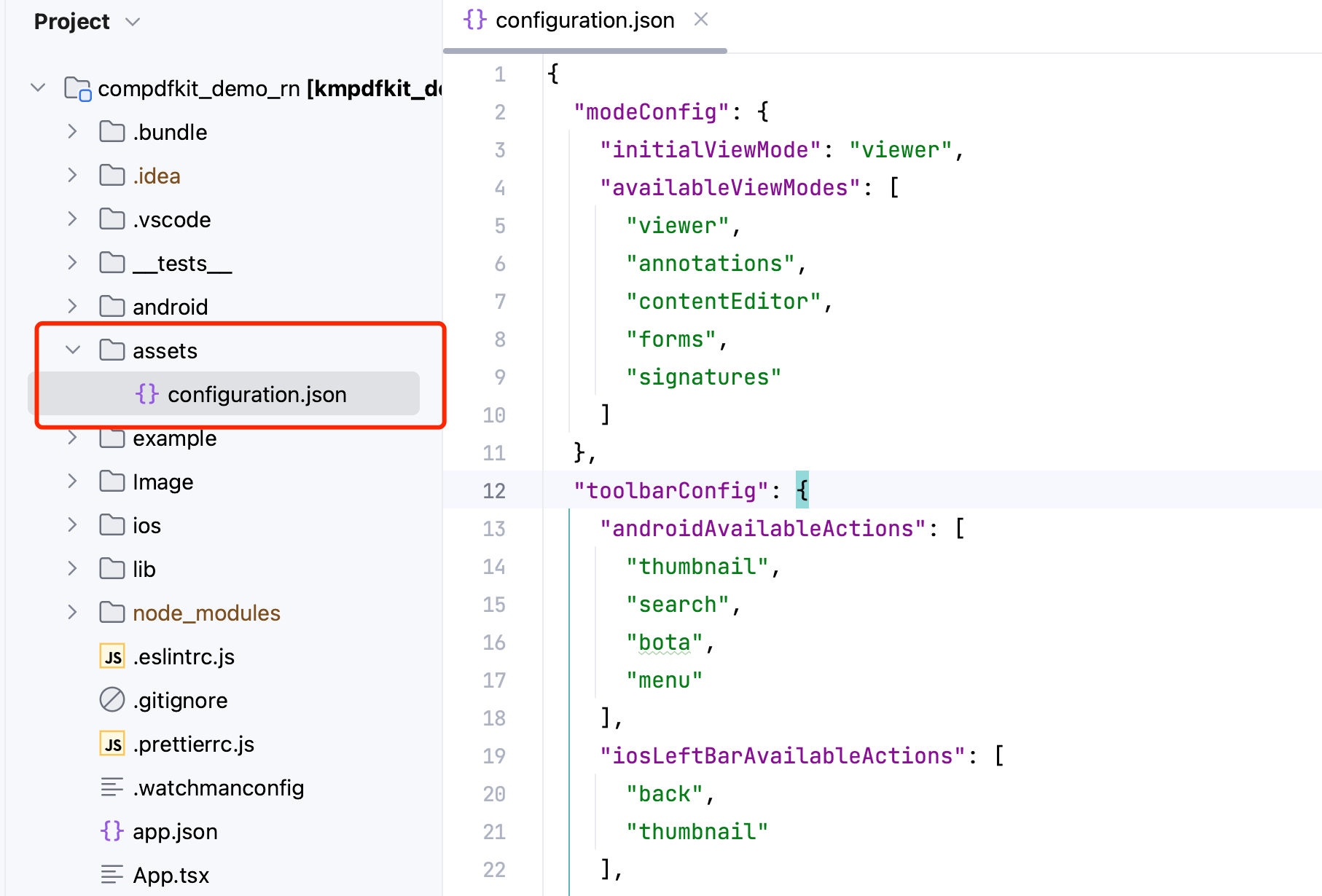
- Open your
App.tsx
file:
bash
open App.tsx
- Replace the entire contents of
App.tsx
with the following code snippet:
js
/**
* Copyright © 2014-2024 PDF Technologies, Inc. All Rights Reserved.
*
* THIS SOURCE CODE AND ANY ACCOMPANYING DOCUMENTATION ARE PROTECTED BY INTERNATIONAL COPYRIGHT LAW
* AND MAY NOT BE RESOLD OR REDISTRIBUTED. USAGE IS BOUND TO THE ComPDFKit LICENSE AGREEMENT.
* UNAUTHORIZED REPRODUCTION OR DISTRIBUTION IS SUBJECT TO CIVIL AND CRIMINAL PENALTIES.
* This notice may not be removed from this file.
*/
import React, { Component } from 'react';
import configuration from './assets/configuration.json';
import DocumentPicker from 'react-native-document-picker'
import {
Platform,
StyleSheet,
Text,
View,
Button,
NativeModules
} from 'react-native';
const instructions = Platform.select({
ios: 'Press Cmd+R to reload,\n' +
'Cmd+D or shake for dev menu',
android: 'Double tap R on your keyboard to reload,\n' +
'Shake or press menu button for dev menu',
});
type Props = {};
export default class App extends Component<Props> {
componentDidMount(){
// Fill in your online license
NativeModules.OpenPDFModule.initialize('your android platform compdfkit license', 'your ios platform compdfkit license')
// Fill in your offline license
// NativeModules.OpenPDFModule.init_('your compdfkit license')
}
render() {
return (
<View style={styles.container}>
<Text style={styles.welcome}>
Welcome to React Native!
</Text>
<Text style={styles.instructions}>
To get started, edit App.tsx
</Text>
<Text style={styles.instructions}>
{instructions}
</Text>
<Button
title={'Open sample document'}
onPress={() => {
this.jumpToNativeView();
}}
/>
<View style={{margin:5}}/>
<Button
title={'pick document'}
onPress={() => {
try {
const pickerResult = DocumentPicker.pick({
type: [DocumentPicker.types.pdf]
});
pickerResult.then(res => {
if (Platform.OS == 'android') {
// only android
NativeModules.OpenPDFModule.openPDFByUri(res[0].uri, '', JSON.stringify(configuration))
} else {
NativeModules.OpenPDFModule.openPDFByConfiguration(res[0].uri, '', JSON.stringify(configuration))
}
})
} catch (err) {
}
}}
/>
</View>
);
}
jumpToNativeView() {
NativeModules.OpenPDFModule.openPDF(JSON.stringify(configuration))
// android: filePath, ios:URL
// NativeModules.OpenPDFModule.openPDFByConfiguration(filePath, password, JSON.stringify(configuration))
// only android platform
// NativeModules.OpenPDFModule.openPDFByUri(uriString, password, JSON.stringify(configuration))
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
}
});
- The app is now ready to launch! Go back to the terminal.
bash
//Run on Android devices
npx react-native run-android
//Run on iOS devices
npx react-native run-ios
We have provided two quick ways to open PDFs:
- Open the document in the specified path
tsx
// The ios platform can use this method to pass in the url string
NativeModules.OpenPDFModule.openPDFByConfiguration(String filePath, String password, String configuration)
- Opening a document using Uri on the Android platform.
tsx
NativeModules.OpenPDFModule.openPDFByUri(String uriString, String password, String configuration)